Variables And Script Editor
The Script Editor provides flexible custom logic capabilities, primarily used in Dynamic Looping Actions and Conditional Scripts. It supports standard Visual Studio Code features. The Script Editor consists of several components:
- Top Panel: Contains the "Run Script" button.
- Left Panel: Houses the "Dynamic Variables JSON" editor, which displays generated examples of dynamic variables passed to the script.
- JS Script Panel: The main area where you can write your Java Script code.
- Output Panel: Located at the bottom, this panel displays the script output.
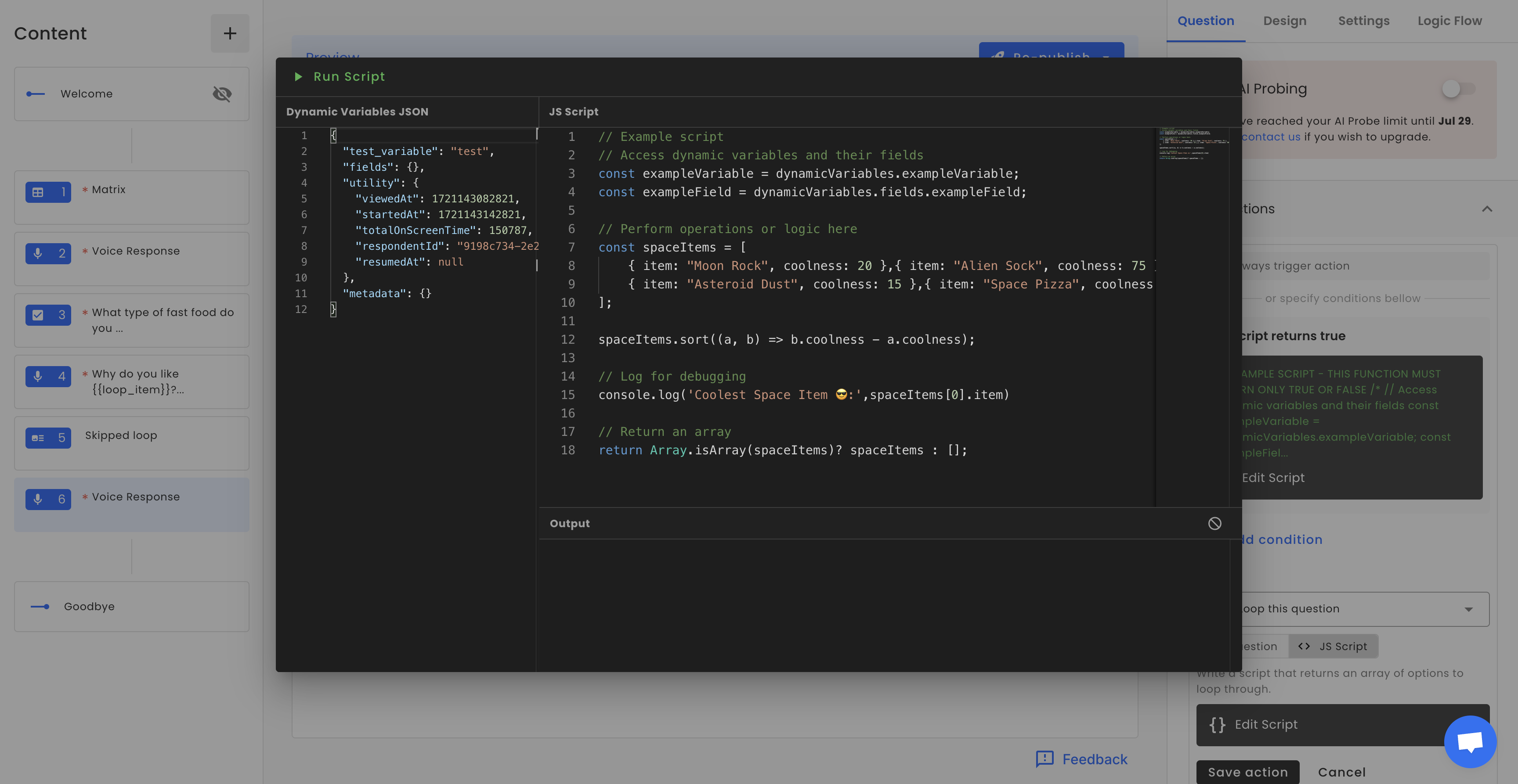
Scripting
To execute the script, click the “Run Script” button located in the top panel. The results of the script will be displayed in the output panel.
Result Output
If the script runs successfully, the output panel will display a RESULT
message with the data that will be passed in the final result.
[2024-07-16T15:59:16.692Z] RESULT: [{"item":"Space Pizza","coolness":90},{"item":"Alien Sock","coolness":75},{"item":"Moon Rock","coolness":20},{"item":"Asteroid Dust","coolness":15}
Logging Output
Logging is useful for debugging purposes. To send log messages to the output, use the console.log() function. The output panel will display logging messages starting with LOG
.
const simpleString = 'Test'
const simpleNumber = 12345
const obj = {'a': 1, 'b': 2};
// custom message
console.log('I love Voiceform!');
// logging a single variable
console.log('Text 1:', simpleString);
// logging multiple variables
console.log('Simple String:', simpleString, 'Simple Number:', simpleNumber);
// logging objects
console.log('My Object:', JSON.stringify(obj, null, 2))
// Return an array
return [];
[2024-07-16T16:11:38.246Z] LOG: I love Voiceform!
[2024-07-16T16:11:38.247Z] LOG: Text 1: Test
[2024-07-16T16:11:38.248Z] LOG: Simple String: Test Simple Number: 12345
[2024-07-16T16:11:38.248Z] LOG: My Object: {
"a": 1,
"b": 2
}
...
Error Output
If the script contains an error or returns an incorrect final result, the output will display an error message.
[2024-07-16T16:16:09.789Z] ERROR: dsdkjsdj is not defined
[2024-07-16T16:17:32.436Z] VALIDATION ERROR: The script should return an array
Dynamic Variables
The "Dynamic Variables JSON" editor provides a generated example of dynamicVariables
that can be passed into the script. This JSON can be edited directly in the panel to customize the dynamic variables as needed. Note that the values are generated examples and might not be exactly the same but will be very similar. In the script, dynamic variables can be accessed through the reserved variable dynamicVariables
. This variable is a JavaScript object, so its properties can be accessed using dot notation, such as dynamicVariables.utility
or dynamicVariables.my_custom_variable
.
Pre-declared Variables
If a dynamic variable has been declared via survey settings, it will be passed to the panel. Additionally, if the variable has a default value, it will be populated accordingly.
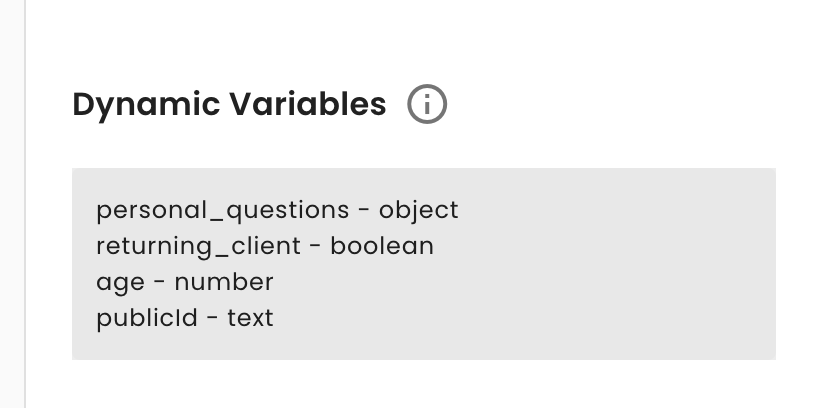
Pre-declared Dynamic Variables in Survey Settings
{
"personal_questions": [
"What is your full name?",
...
],
"returning_client": false,
"age": 18,
"publicId": null,
"fields": {...},
"utility": {...},
"metadata": {...}
}
/**
* Script Example: Select 5 Random Personal Questions
*
* Description:
* This script selects 5 random questions from the list of personal questions
* provided in the `dynamicVariables.personal_questions`. The script shuffles
* the array of questions using the Fisher-Yates algorithm, selects the first
* 5 questions from the shuffled array, and returns the selected questions.
*/
// Extract the list of personal questions from dynamic variables
const { personal_questions } = dynamicVariables;
// Function to shuffle an array using the Fisher-Yates algorithm
function shuffleArray(array) {
for (let i = array.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[array[i], array[j]] = [array[j], array[i]];
}
return array;
}
// Shuffle the personal questions array
const shuffledQuestions = shuffleArray(personal_questions);
// Select the first 5 questions from the shuffled array
const selectedQuestions = shuffledQuestions.slice(0, 5);
// Return the selected questions
return selectedQuestions;
Fields Variables
If a variable has been assigned to a specific question via question fields, it will be populated under the fields
object. An example of how the value of this variable might look will be generated, but keep in mind that this is just an example. The actual values are expected to differ when the survey is live.
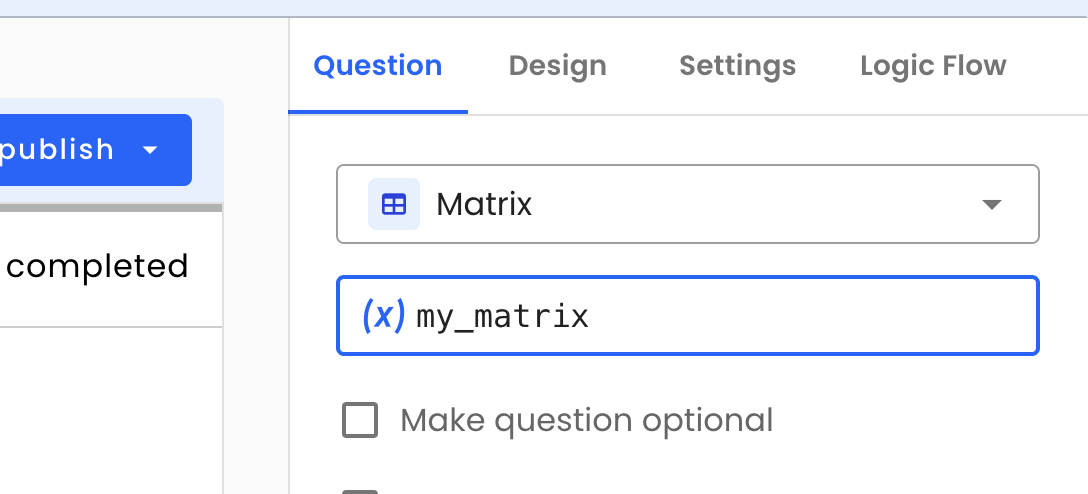
{
...
"fields": {
"my_matrix": {
"row_ef268bd3aa0749839b27190582d49c42": {
"default_radio": {
"col_id": "column_e315f5a093fe414f9659104421645b63",
"col_title": "Column 4",
"row_title": "Row 1",
"type": "radio",
"value": "Column 4"
}
},
"row_7d306adfa07240eeab14ea4ce425e8f2": {
"default_radio": {
"col_id": "column_46834df5539340dba01e91e5db192977",
"col_title": "Column 1",
"row_title": "Row 2",
"type": "radio",
"value": "Column 1"
}
},
"row_b45ccb93a1d24f1887ebee7fd4ea8a05": {
"default_radio": {
"col_id": "column_46834df5539340dba01e91e5db192977",
"col_title": "Column 1",
"row_title": "Row 3",
"type": "radio",
"value": "Column 1"
}
}
}
},
"utility": {...},
"metadata": {...}
}
Utility Variables
Utility variables are set by Voiceform when a respondent opens the survey or while they are filling it out.
Variable Name | Description | Default Value |
---|---|---|
viewedAt | A timestamp set when the survey is opened, in milliseconds since the epoch. E.g.1721143082821 | null |
startedAt | A timestamp set when the first answer is provided in the survey, in milliseconds since the epoch. E.g. 1721143142821 | null |
totalOnScreenTime | The number of milliseconds the user has spent on the survey page. Time spent on a different screen or browser tab is not included, except for time during audio recording. E.g. 150787 | 0 |
respondentId | A unique public UUID of the respondent. E.g.9198c734-2e29-4138-b109-f5adfd2fe259 | null |
resumedAt | A timestamp set if the user resumes a previously saved survey, in milliseconds since the epoch. 1721143102821 | null |
Metadata Variables
Metadata variables are parameters passed to the survey via query parameters, plus information from question types like "Email", "Phone", and "Name" (metadata.email
, metadata.phoneNumber
, metadata.name
). You can use metadata variables instead of declaring dynamic variables if you are fine with the variable being a string
.
For the survey link: https://app.voiceform.com/[survey_hash]/?customId=12345678&existingClient=true
{
...
"metadata": {
"customId": "12345678",
"existingClient": "true"
}
...
}
// Skipping email question if it's an existing customer
const { metadata } = dynamicVariables;
// Log for debugging
console.log('Metadata Custom ID:', metadata.customId);
if (metadata.customId && metadata.existingClient === 'true') {
// We skip this question
return true;
}
// We need to request an email
return false;
Updated about 1 month ago