Mobile Apps
This document provides a comprehensive guide for integrating Voiceform surveys into mobile applications, with step-by-step examples for React Native, iOS (Swift), and Android (Kotlin) implementations using WebView components.
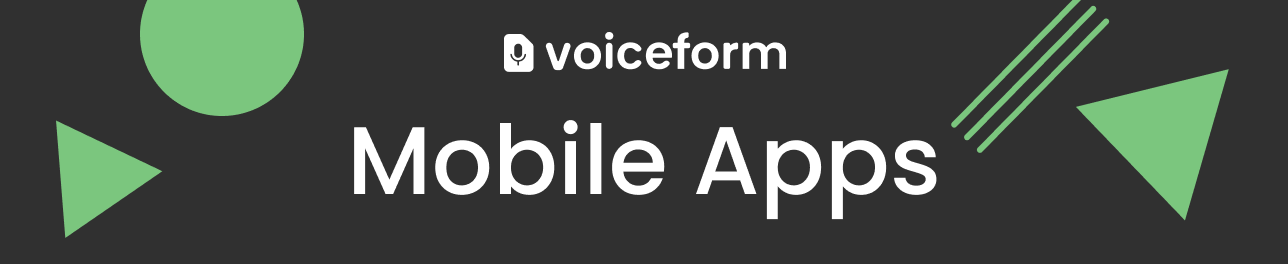
Voiceform Mobile App Integration Guide
Voiceform surveys can be seamlessly integrated into mobile applications using a WebView component. This guide will provide you with the necessary steps and code examples for integrating Voiceform into your mobile app on various platforms, including React Native, iOS, and Android.
Basic Integration Flow
- Create a Voiceform Survey: Start by creating your survey on Voiceform.
- Navigate to the Distribute Page: Once your survey is ready, go to the "Distribute" page within the Voiceform dashboard.
- Copy the Survey Link: Copy the link provided for your survey (e.g.,
<https://app.voiceform.com/to/9V7GKLwJs2jkBbMt>
). This link will be used in the WebView component within your mobile app. Optionally, you may append metadata or dynamic variables to this link as needed.
React Native Example
Below is an example of how to integrate a Voiceform survey into a React Native app using a modal to display the survey within a WebView component.
// ./components/Voiceform.js
import React from 'react';
import { Modal, SafeAreaView, View, Text, TouchableOpacity, StyleSheet } from 'react-native';
import { WebView } from 'react-native-webview';
const Voiceform = ({ modalVisible, setModalVisible }) => {
const onMessage = (event) => {
try{
// Check if the message from the WebView is 'submitted'
if (event.nativeEvent.data) {
const data = JSON.parse(event.nativeEvent.data)
if(data && data.type && data.type === 'voiceform.form-submitted'){
setModalVisible(false); // Close the survey
}
}
}catch(e){
console.error(e);
}
};
return (
<Modal
animationType="slide"
transparent={false}
visible={modalVisible}
>
<SafeAreaView style={{ flex: 1 }}>
<View style={styles.modalHeader}>
<Text style={styles.headerText}>Feedback Form</Text>
<TouchableOpacity style={styles.closeButton} onPress={() => setModalVisible(false)}>
<Text style={styles.closeButtonText}>Close</Text>
</TouchableOpacity>
</View>
<WebView
source={{ uri: 'https://app.voiceform.com/to/9V7GKLwJs2jkBbMt' }} // Replace with your Voiceform survey link
onMessage={onMessage}
style={styles.webview}
/>
</SafeAreaView>
</Modal>
);
};
// Define your styles
const styles = StyleSheet.create({
modalHeader: {
padding: 16,
backgroundColor: '#f8f8f8',
flexDirection: 'row',
justifyContent: 'space-between',
alignItems: 'center',
},
headerText: {
fontSize: 18,
fontWeight: 'bold',
},
closeButton: {
padding: 8,
backgroundColor: '#007AFF',
borderRadius: 4,
},
closeButtonText: {
color: '#fff',
fontSize: 14,
},
webview: {
flex: 1,
},
});
export default Voiceform;
Live Demo
You can view a live implementation of this integration by exploring the interactive example below:
iOS (Swift) Example
Below is an example of how to integrate a Voiceform survey into an iOS app using Swift and a WebView component.
import UIKit
import WebKit
class VoiceformViewController: UIViewController, WKScriptMessageHandler {
var webView: WKWebView!
override func viewDidLoad() {
super.viewDidLoad()
let contentController = WKUserContentController()
contentController.add(self, name: "voiceform")
let config = WKWebViewConfiguration()
config.userContentController = contentController
webView = WKWebView(frame: self.view.frame, configuration: config)
webView.navigationDelegate = self as? WKNavigationDelegate
self.view.addSubview(webView)
// Load the Voiceform survey
if let url = URL(string: "https://app.voiceform.com/to/9V7GKLwJs2jkBbMt") {
let request = URLRequest(url: url)
webView.load(request)
}
}
// This function handles the messages received from the WebView
func userContentController(_ userContentController: WKUserContentController, didReceive message: WKScriptMessage) {
if message.name == "voiceform" {
// Attempt to parse the message body as a JSON object
if let messageBody = message.body as? [String: Any],
let type = messageBody["type"] as? String,
type == "voiceform.form-submitted" {
// Handle form submission, e.g., close the view controller
self.dismiss(animated: true, completion: nil)
}
}
}
}
In this iOS implementation, a WKWebView
is used to load the Voiceform survey, and a WKScriptMessageHandler
is implemented to handle messages from the WebView, such as when the form is submitted.
Android (Kotlin) Example
Below is an example of how to integrate a Voiceform survey into an Android app using Kotlin and a WebView component.
import android.os.Bundle
import android.webkit.JavascriptInterface
import android.webkit.WebResourceRequest
import android.webkit.WebView
import android.webkit.WebViewClient
import androidx.appcompat.app.AppCompatActivity
class VoiceformActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_voiceform)
val webView: WebView = findViewById(R.id.webview)
// Enable JavaScript in the WebView
webView.settings.javaScriptEnabled = true
// Set a custom WebViewClient
webView.webViewClient = object : WebViewClient() {
override fun shouldOverrideUrlLoading(view: WebView?, request: WebResourceRequest?): Boolean {
return false
}
override fun onPageFinished(view: WebView?, url: String?) {
// Inject JavaScript to listen for the form submission event
view?.evaluateJavascript(
"""
(function() {
document.body.addEventListener('voiceform.form-submitted', function() {
Android.onFormSubmitted();
});
})();
""".trimIndent(),
null
)
}
}
// Add a JavaScript interface to allow JavaScript to communicate with Android
webView.addJavascriptInterface(object {
@JavascriptInterface
fun onFormSubmitted() {
// Handle form submission by finishing the activity
runOnUiThread {
finish()
}
}
}, "Android")
// Load the Voiceform survey
webView.loadUrl("https://app.voiceform.com/to/9V7GKLwJs2jkBbMt")
}
}
In this Android implementation, a WebView
is used to load the Voiceform survey, and JavaScript is enabled to handle form submissions. The JavascriptInterface
is used to bridge JavaScript and Kotlin, allowing the app to respond to the form submission event.
Updated 3 months ago